【Python】bitflyer の Private API を使って資産情報を取得してみる
おはようございます。
昨日に引き続き、Bitflyer の API をいじりたいと思います。
今回は Private API を用いて、個人の情報を取得します。
Bitflyer のアカウントが必要となりますので、お持ちでない方はまずアカウントの作成をお願いします。
プログラムは前回のものを流用します。
【Python】pubnub を使って bitflyer のTicker情報をリアルタイム表示する
スポンサーリンク
APIキーの取得
bitflyer にログインし、BitFlyer Lightning の画面を表示したら、左側のサイドメニューを開いて「API」メニューを選択します。
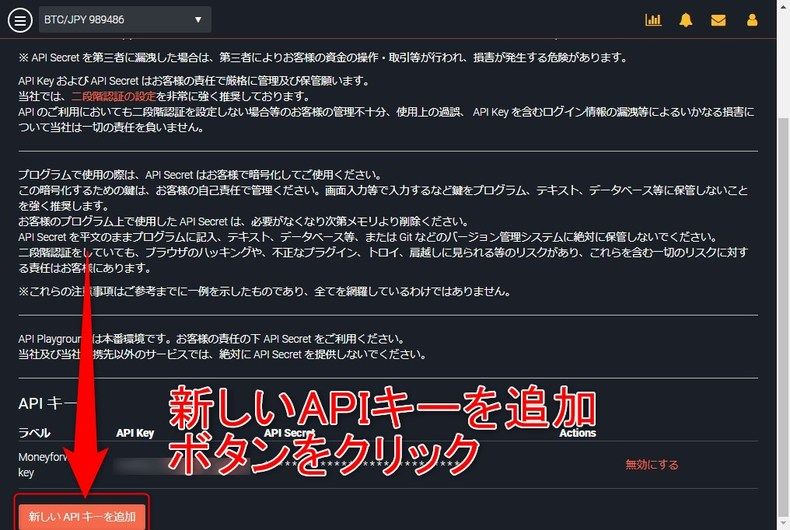
「新しいAPIキーを追加」ボタンをクリックします。
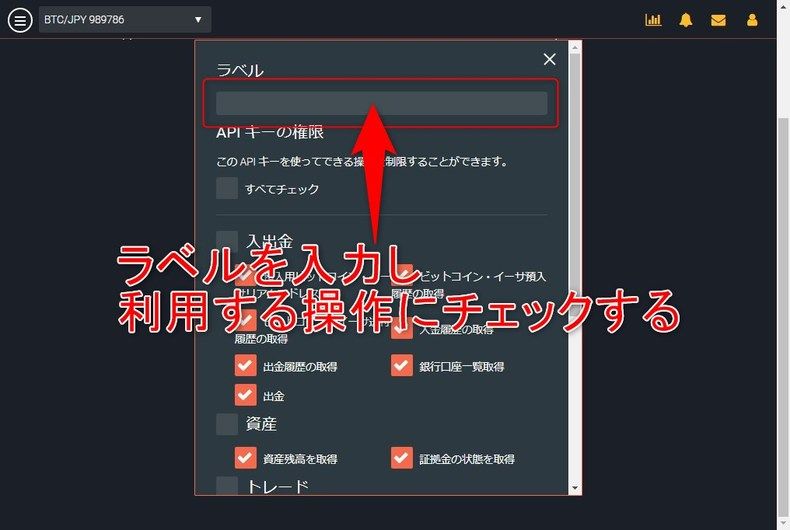
APIキーのラベルを入力、利用する操作にチェックをしたら、下部にある「OK」ボタンをクリックします。
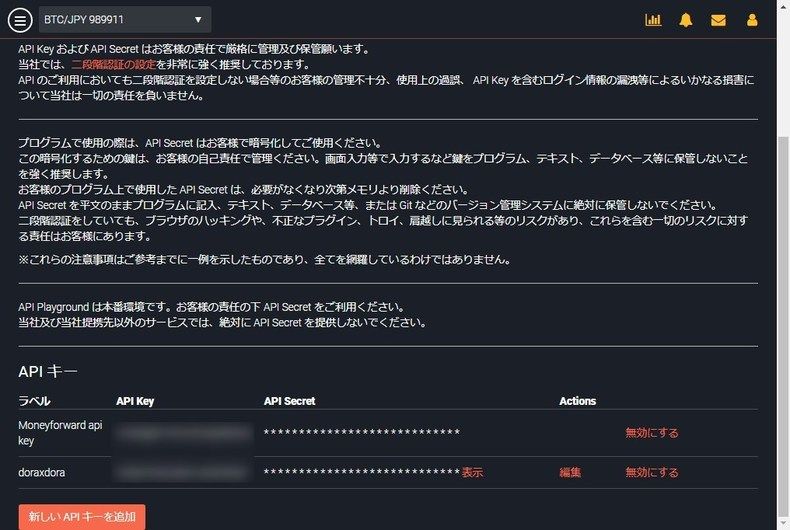
APIキーが追加されるので、「API Key」と「API Secret」を控えます。
※注意書きにもありますが、第3者の目に触れないような管理を、自己責任でお願いします。
画面の修正
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | <!DOCTYPE html> <html> <head> <title>{{ title }}</title> <link rel="stylesheet"href="{{ static_url('css/style.css') }}"/> <script type="text/javascript"src="{{ static_url('js/script.js') }}"></script> <script type="text/javascript"src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min.js"></script> </head> <body onload="initialize();"> <div id="container"> <div style="clear:both; padding-top:10px;"> <table id="tickerTable"> <tr id="header"> <th style="width:5%">種別</th> <th style="width:10%">時刻</th> <th style="width:5%">ID</th> <th style="width:5%">売値</th> <th style="width:5%">買値</th> <th style="width:10%">売り数量</th> <th style="width:10%">買い数量</th> <th style="width:10%">売り注文総数</th> <th style="width:10%">買い注文総数</th> <th style="width:10%">最終取引価格</th> <th style="width:10%">出来高</th> <th style="width:10%">価格単位出来高</th> </tr> </table> </div> <div style="clear:both; padding-top:10px;"> <input type="button"value="更新"onclick="updateBalance();" /> <table id="balanceTable"> <tr><th>円</th><td id="jpy"></td></tr> <tr><th>ビットコイン</th><td id="btc"></td></tr> <tr><th>ビットコインキャッシュ</th><td id="bch"></td></tr> <tr><th>イーサ</th><td id="eth"></td></tr> <tr><th>イーサクラシック</th><td id="etc"></td></tr> <tr><th>ライトコイン</th><td id="ltc"></td></tr> <tr><th>モナコイン</th><td id="mona"></td></tr> <tr><th>リスク</th><td id="lsk"></td></tr> </table> </div> </div> </body> </html> |
style.css
新規追加したテーブルのスタイルを追加
1 2 3 4 5 6 7 | #balanceTable { width:400px; } #balanceTable th { padding-left:5px; text-align:left; } |
プログラムの修正
script.js
Tickerのテーブルは最大10行までとし、新しく追加したボタンの処理を追加します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 | // スクリプト読み込み時の処理 functioninitialize(){ addEmptyRow(10); updateBalance(); varconnection=newWebSocket('ws://127.0.0.1:8888/ticker'); connection.onmessage=function(e){ vardata=JSON.parse(e.data.replace(/\\/g,"")); vartable=$("#tickerTable"); // 日付け変換 vardate=newDate(data.timestamp); data.timestamp=date.toLocaleString(); // テーブルに追加 vartr=document.createElement("tr"); $.each(data,function(i,cell){ vartd=document.createElement("td"); td.innerHTML=cell; tr.appendChild(td); }); varrows=table.find("tr"); if(rows.length>10){ $("#tickerTable tr:last").remove(); } $(tr).insertAfter("#header"); }; } /** * 空行をテーブルに追加します */ functionaddEmptyRow(rowCount){ for(i=0;i<rowCount;i++){ vartr=document.createElement("tr"); for(j=0;j<12;j++){ vartd=document.createElement("td"); tr.appendChild(td); } $(tr).insertAfter("#header"); } } /** * 資産情報を更新します. */ functionupdateBalance(){ $.ajax({ url:"http://localhost:8888/balance", type:"POST", success:function(jsonResponse){ jsonResponse=jsonResponse.replace(/\\/g,""); vardata=JSON.parse(jsonResponse); for(row indata){ switch(data[row].currency_code){ case"JPY": $("#jpy").text(data[row].amount); break; case"BTC": $("#btc").text(data[row].amount); break; case"BCH": $("#bch").text(data[row].amount); break; case"ETH": $("#eth").text(data[row].amount); break; case"ETC": $("#etc").text(data[row].amount); break; case"LTC": $("#ltc").text(data[row].amount); break; case"MONA": $("#mona").text(data[row].amount); break; case"LSK": $("#lsk").text(data[row].amount); break; default: console.log("該当なし"); break; } } }, error:function(){ } }); } |
Sample.py
資産情報を取得するURLマッピングの処理を追加
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 | importhmac importhashlib access_key="API キー" secret_key="シークレットキー" classGetBalanceHandler(tornado.web.RequestHandler): u""" メイン処理 """ definitialize(self): logging.info("initialize") defpost(self): globalsecret_key globalaccess_key timeout=None method="GET" access_time=str(time.time()) endpoint="/v1/me/getbalance" # body = "?" + urllib.parse.urlencode(params) encode_secret_key=str.encode(secret_key) encode_text=str.encode(access_time+method+endpoint) access_sign=hmac.new(encode_secret_key,encode_text,hashlib.sha256).hexdigest() auth_header={ 'ACCESS-KEY':access_key, 'ACCESS-TIMESTAMP':access_time, 'ACCESS-SIGN':access_sign, 'Content-Type':'application/json' } url="https://api.bitflyer.jp"+endpoint withrequests.Session()ass: ifauth_header: s.headers.update(auth_header) ifmethod=="GET": response=s.get(url,params=None,timeout=timeout) else: # method == "POST": response=s.post(url,data=None,timeout=timeout) iflen(response.content)>0: content=json.loads(response.content.decode("utf-8")) logging.info(content) self.write(json.dumps(content,ensure_ascii=False)) app=tornado.web.Application([ (r"/",MainHandler), (r"/ticker",SendWebSocket), (r"/balance",GetBalanceHandler) ], template_path=os.path.join(os.getcwd(),"templates"), static_path=os.path.join(os.getcwd(),"static"), js_path=os.path.join(os.getcwd(),"js"), ) |
起動してみる
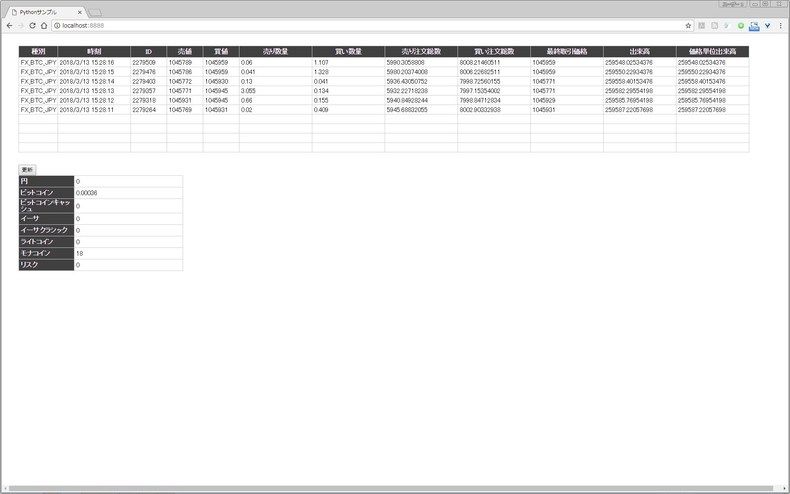
起動時にとりあえず取得してテーブルに設定するような形にしてあります。
Private API はアクセスの回数など、制限があるためむやみに繰り返し処理をしない方が無難です。
まとめ
あまり使いどころはなさそうですが、とりあえず資産の状況を取得・表示することができました。
次回は注文関係をやってみれたらと思います。
ではでは。
ディスカッション
コメント一覧
まだ、コメントがありません