【Python】FullCalendar を使ったWEBページを作ってみる
おはようございます。
ちょっと前に、JQueryプラグインの「FullCalendar.js」を使って
カレンダーを表示するサンプルを作ってみましたが、
今回は PythonでWEBサーバーを立てて、実際にサーバーとやりとりするサンプルを作ってみました。
FullCalendarの記事はこちら。
スポンサーリンク
プロジェクトの作成
PyCharmを利用していきます。
プロジェクトの作成方法は次の記事を参考にしていただければ。
プロジェクトの構成は次の通り。
CalendarSample
│ Main.py
│
├─static
│ ├─css
│ │ fullcalendar.min.css
│ │ fullcalendar.print.min.css
│ │ style.css
│ │
│ ├─js
│ │ fullcalendar.min.js
│ │ moment.min.js
│ │
│ └─lang
│ ja.js
│
└─templates
index.html
パッケージの追加
Tornadoを利用します。
次の記事を参考にパッケージを追加してください。
プログラム
画面
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 | <!DOCTYPE html> <html> <head> <title>カレンダーサンプル</title> <link rel="stylesheet"href="{{ static_url('css/fullcalendar.min.css') }}"/> <link rel="stylesheet"href="{{ static_url('css/style.css') }}"/> <script type="text/javascript"src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min.js"></script> <script type="text/javascript"src="{{ static_url('js/moment.min.js') }}"></script> <script type="text/javascript"src="{{ static_url('js/fullcalendar.min.js') }}"></script> <script type="text/javascript"src="{{ static_url('lang/ja.js') }}"></script> <script> // ページ読み込み時の処理 $(document).ready(function(){ // カレンダーの設定 $('#calendar').fullCalendar({ height:550, lang:"ja", header:{ left:'prev,next today', center:'title', right:'month,basicWeek,basicDay' }, selectable:true, selectHelper:true, navLinks:true, events:{ url:'http://localhost:8080/getCalendar', error:function(){ $('#script-warning').show(); } }, select:function(start,end){ vartitle=prompt("予定タイトル:"); vareventData; if(title){ eventData={ title:title, start:start, end:end }; $('#calendar').fullCalendar('renderEvent',eventData,true); } $('#calendar').fullCalendar('unselect'); }, editable:true, eventLimit:true, }); }); </script> </head> <body> <div id='calendar'></div> </body> </html> |
style.css
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 | body { margin:40px10px; padding:0; font-family:"Lucida Grande",Helvetica,Arial,Verdana,sans-serif; font-size:14px; } #calendar { max-width:900px; margin:0auto; } html > body { font-family:'Noto Sans Japanese','SegoeUI','Verdana','Helvetica','Arial',sans-serif; } .fc-left h2 { font-weight:normal; } .fc-widget-header th { background-color:#f8dbc6; } th.fc-day-header, th.fc-widget-header { color:#550000; font-size:14px; font-weight:normal; } .fc-sun > span { color:#cd384b; } .fc-sat > span { color:#415ea6; } td.fc-sun { background-color:#fae6e3; } td.fc-sat { background-color:#dceef7; } .fc-unthemed .fc-content, .fc-unthemed .fc-divider, .fc-unthemed .fc-list-heading td, .fc-unthemed .fc-list-view, .fc-unthemed .fc-popover, .fc-unthemed .fc-row, .fc-unthemed tbody, .fc-unthemed td, .fc-unthemed th, .fc-unthemed thead { border-color:#d1ccd9; } .fc-ltr .fc-basic-view .fc-day-top .fc-day-number { float:left; } |
サーバー側
とりあえずデータはべた書きですが。
Main.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 | importjson importlogging importos importtornado.ioloop fromtornado.web importRequestHandler fromtornado.options importoptions classMainHandler(tornado.web.RequestHandler): defget(self): self.render("index.html") classGetCalendar(RequestHandler): """ カレンダー取得 """ definitialize(self): logging.info("GetCalendar [initialize]") defget(self): logging.info("GetCalendar [get]") data=[ { "title":"All Day Event", "start":"2018-06-01" }, { "title":"Long Event", "start":"2018-06-07", "end":"2018-06-10" }, { "id":"999", "title":"Repeating Event", "start":"2018-06-09T16:00:00-05:00" }, { "id":"999", "title":"Repeating Event", "start":"2018-06-16T16:00:00-05:00" }, { "title":"Conference", "start":"2018-06-11", "end":"2018-06-13" }, { "title":"Meeting", "start":"2018-06-12T10:30:00-05:00", "end":"2018-06-12T12:30:00-05:00" }, { "title":"Lunch", "start":"2018-06-12T12:00:00-05:00" }, { "title":"Meeting", "start":"2018-06-12T14:30:00-05:00" }, { "title":"Happy Hour", "start":"2018-06-12T17:30:00-05:00" }, { "title":"Dinner", "start":"2018-06-12T20:00:00" }, { "title":"Birthday Party", "start":"2018-06-13T07:00:00-05:00" }, { "title":"Click for Google", "url":"http://google.com/", "start":"2018-06-28" } ] self.write(json.dumps(data)) app=tornado.web.Application([ (r"/",MainHandler), (r"/getCalendar",GetCalendar), ], template_path=os.path.join(os.getcwd(),"templates"), static_path=os.path.join(os.getcwd(),"static"), ) if__name__=="__main__": options.parse_command_line() app.listen(8080) logging.info("server started") tornado.ioloop.IOLoop.instance().start() |
起動してみる
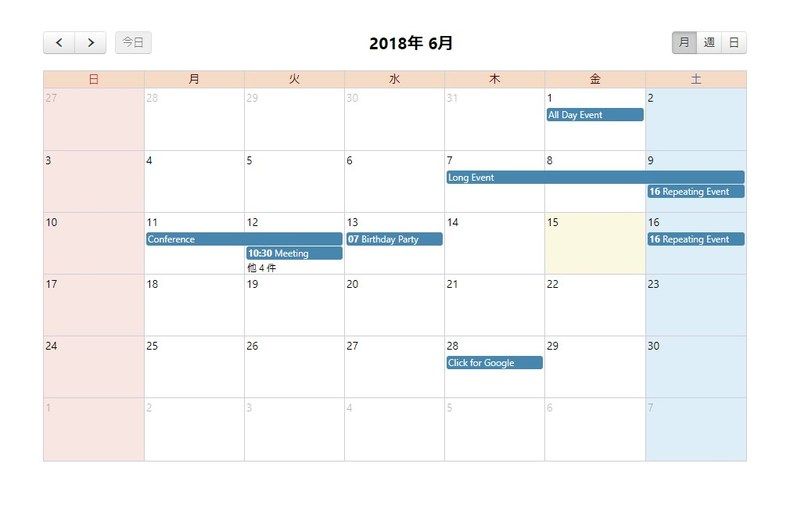
前回のサンプルは月間のみでしたが、週表示、日表示もついでにやってみました。
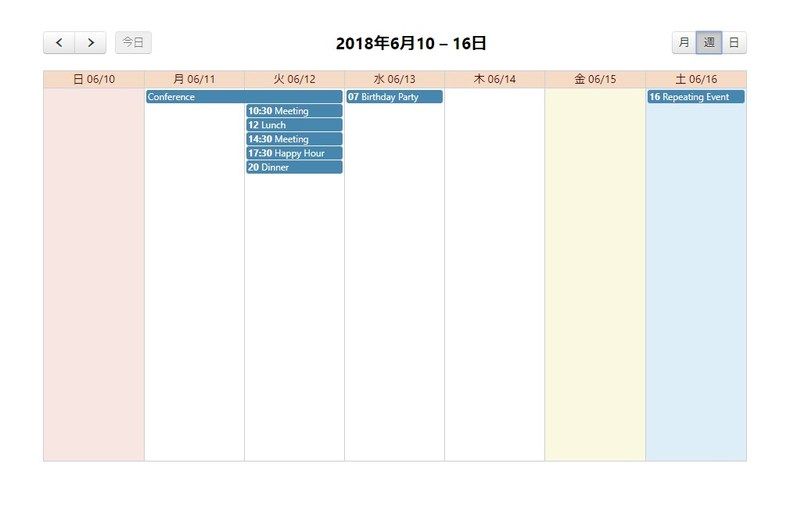
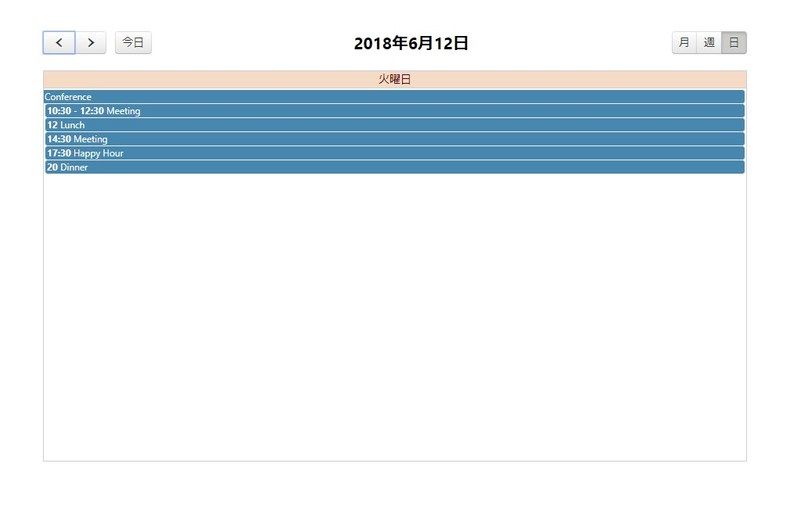
まとめ
ひとまずこれでサーバー側とのやり取りができるようになりました。
次回は実際にデータベースを用いてデータの登録までやりたいと思います。
ではでは。
ディスカッション
コメント一覧
まだ、コメントがありません